Api Authentication for Laravel
In this post I will be looking at using the different packages available to register & authenticate your users on a Laravel setup designed as a pure API implementation
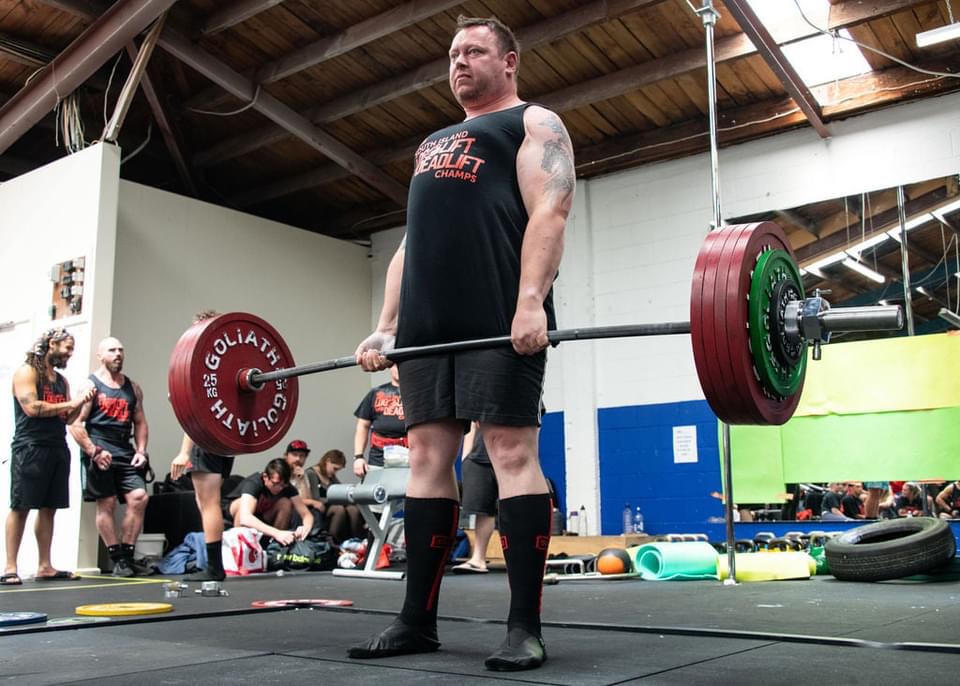
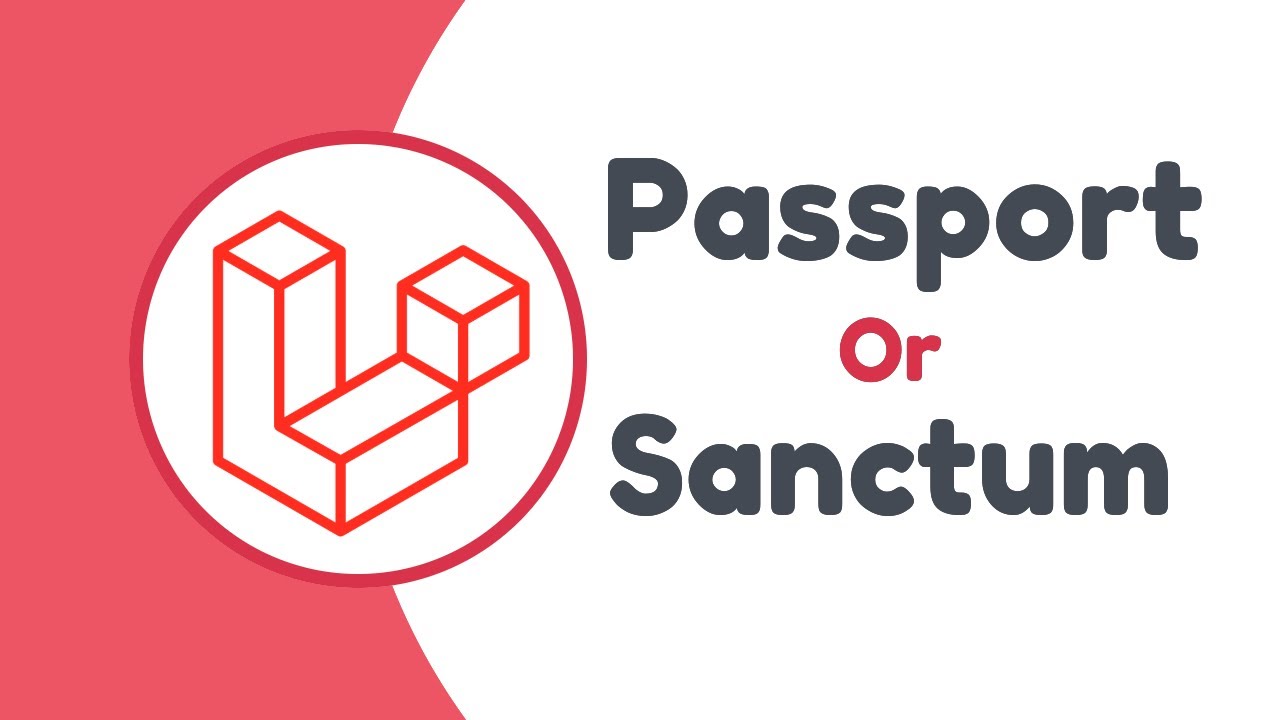
Laravel offers a few core systems for authentication dependant on your requirements these are as follows
Laravel Fortify
Laravel Fortify is a frontend agnostic authentication backend implementation for Laravel. Fortify registers the routes and controllers needed to implement all of Laravel's authentication features, including login, registration, password reset, email verification, and more. After installing Fortify, you may run the route:list
Artisan command to see the routes that Fortify has registered.
Since Fortify does not provide its own user interface, It is a good package to consider when doing auth management through the API
Laravel Breeze
Laravel Breeze is a minimal, simple implementation of all of Laravel's authentication features, including login, registration, password reset, email verification, and password confirmation. Laravel Breeze's default view layer is made up of simple Blade templates styled with Tailwind CSS. Breeze provides a wonderful starting point for beginning a fresh Laravel application.
Breeze is more geared towards a frontend blade driven app and as such not a good contender for the API driven system.
Laravel Jetstream
While Laravel Breeze provides a simple and minimal starting point for building a Laravel application, Jetstream augments that functionality with more robust features and additional frontend technology stacks. For those brand new to Laravel, we recommend learning the ropes with Laravel Breeze before graduating to Laravel Jetstream.
Jetstream provides a beautifully designed application scaffolding for Laravel and includes login, registration, email verification, two-factor authentication, session management, API support via Laravel Sanctum, and optional team management. Jetstream is designed using Tailwind CSS and offers your choice of Livewire or Inertia.js driven frontend scaffolding.
Laravel Passport
Laravel Passport provides a full OAuth2 server implementation for your Laravel application in a matter of minutes. Passport is built on top of the League OAuth2 server that is maintained by Andy Millington and Simon Hamp.
Laravel Sanctum
Laravel Sanctum provides a featherweight authentication system for SPAs (single page applications), mobile applications, and simple, token based APIs. Sanctum allows each user of your application to generate multiple API tokens for their account. These tokens may be granted abilities / scopes which specify which actions the tokens are allowed to perform.
Passport Or Sanctum?
Before getting started, you may wish to determine if your application would be better served by Laravel Passport or Laravel Sanctum. If your application absolutely needs to support OAuth2, then you should use Laravel Passport.
However, if you are attempting to authenticate a single-page application, mobile application, or issue API tokens, you should use Laravel Sanctum. Laravel Sanctum does not support OAuth2; however, it provides a much simpler API authentication development experience.
Ok, Boring discussion over, lets get started:
For our purposes we are going to look at installing the following stack: Fortify and Sanctum aswell as Fortify and Passport. You will note that they are quite quickly interchangeable and could even be setup as Fortify, Sanctum and Passport together.
Steps
Install Fortify
Installing fortify you can follow the instructions on the Laravel Documentation Site or as a quick overview, install via composer, publish and migrate.
composer require laravel/fortify
php artisan vendor:publish --provider="Laravel\Fortify\FortifyServiceProvider"
php artisan migrate
you will now find a new folder in your app folder called Actions. This folder will be accessed shortly and deals with our common actions going forward.
Lets look at our config file located at config/fortify.php - I will make note of suggested settings below
...
/*
|--------------------------------------------------------------------------
| Register View Routes
|--------------------------------------------------------------------------
|
| Here you may specify if the routes returning views should be disabled as
| you may not need them when building your own application. This may be
| especially true if you're writing a custom single-page application.
|
*/
'views' => false, //we are using a SPA and as such no views usign blade will be used.
/*
|--------------------------------------------------------------------------
| Features
|--------------------------------------------------------------------------
|
| Some of the Fortify features are optional. You may disable the features
| by removing them from this array. You're free to only remove some of
| these features or you can even remove all of these if you need to.
|
*/
'features' => [
Features::registration(),
Features::resetPasswords(),
Features::emailVerification(),
/* Features::updateProfileInformation(),
Features::updatePasswords(),
Features::twoFactorAuthentication([
'confirmPassword' => true,
]),*/
],
You will note that i commented out a few of the features, we will go over those later in this document.
The vendor:publish command discussed above will also publish the App\Providers\FortifyServiceProvider class. You should ensure this class is registered within the providers array of your application's config/app.php configuration file.
You now have the Register, Reset and email verification endpoints available to your api calls. however will keep getting told CSRF mismatch. There are 2 fixes for this - you can add '/auth/*' to the VerifyCsrfToken middleware or use the Sanctum setup from below - calling '/sanctum/csrf-cookie' to get the csrf and passing it back to the register endpoint.
now you can modifiy this if you want to - say not automatically login when registering, simply add your own controller extending the 'Laravel\Fortify\Http\Controllers\RegisteredUserController' and override the store method and add a new route to that endpoint - disable the one in the fortify config file.
Install Sanctum
Installing sanctum you can follow the instructions on the Laravel Documentation Site or as a quick overview, install via composer, publish and migrate.
composer require laravel/sanctum
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
php artisan migrate
To begin issuing tokens for users, your User model should use the Laravel\Sanctum\HasApiTokens trait:
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable;
}
Next follow the instructions at https://laravel.com/docs/8.x/sanctum#spa-authentication to setup your SPA settings.