Quick Setup of Laravel with Docksal
In this tutorial I will show you how I boilerplate my initial workflows for laravel.
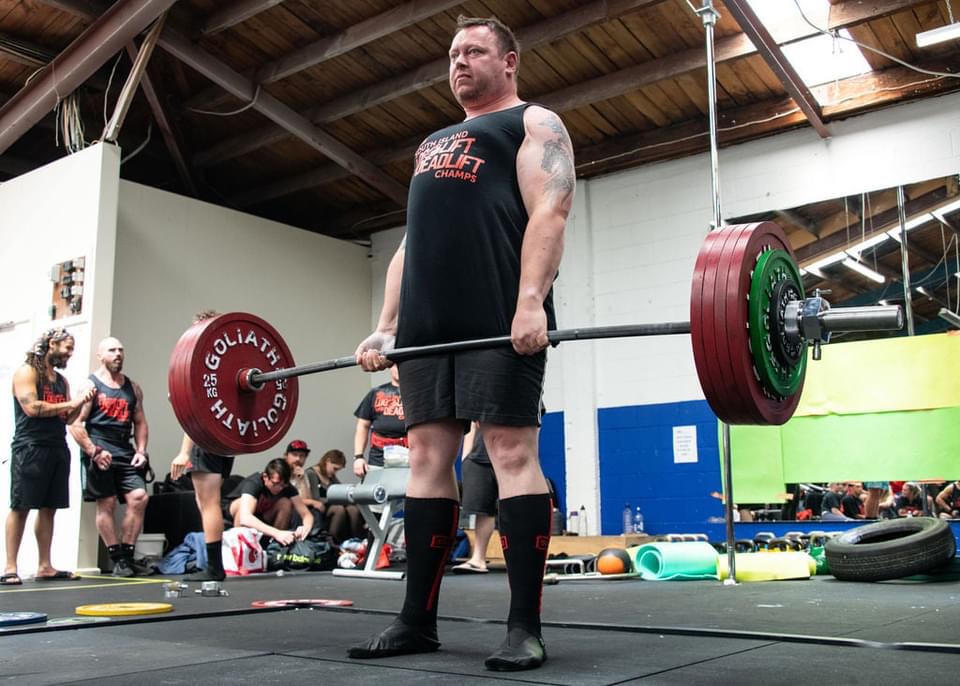
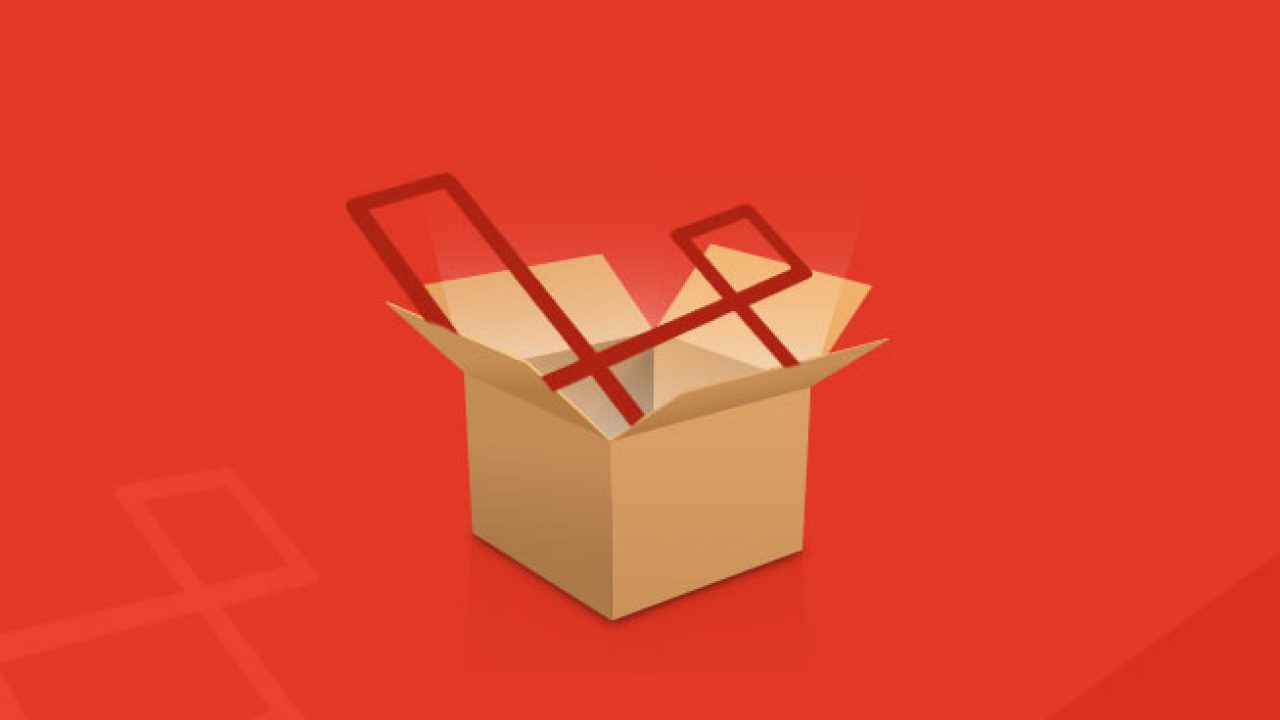
To get started - make sure you have Docksal Installed. Then add the following plugins to your global Docksal environment to make life easier.
fin addon install --global mkcert
fin addon install --global artisan
Thats it. now on to setting up your first site (adjust to your requirements)
cd /Projects # or whereever your containing folders will live
mkdir my-first-site
cd my-first-site
fin rc --image=docksal/cli:stable-php7.4 composer create-project laravel/laravel .
fin config generate --docroot=public
fin config set DOCKSAL_STACK=default
fin config set DB_IMAGE=docksal/db:mysql-5.7
fin config set CLI_IMAGE=docksal/cli:stable-php7.4
fin mkcert create
Laravel is now checked out and the docksal images setup to php 7.4 and mysql 5.7, you can set these to other options which are available
to find these run the following commands:
#retrieves db images
fin image registry docksal/db
#retrieves cli images
fin image registry docksal/cli
Now that we have that setup, lets configure our environment: edit the .docksal/docksal.yml file with the following
version: "2.1"
services:
redis:
extends:
file: ${HOME}/.docksal/stacks/services.yml
service: redis
mail:
extends:
file: ${HOME}/.docksal/stacks/services.yml
service: mail
Next edit your .env file and set your variables:
...
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=default
DB_USERNAME=user
DB_PASSWORD=user
...
REDIS_HOST=redis
REDIS_PASSWORD=null
REDIS_PORT=6379
MAIL_MAILER=smtp
MAIL_HOST=mail
MAIL_PORT=1025
MAIL_USERNAME=null
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
MAIL_FROM_ADDRESS=test@deve.docksal
MAIL_FROM_NAME="${APP_NAME}"
Ok now we are ready. lets do some basic configuration next: run the following
fin start
fin artisan key:generate
fin artisan migrate
fin artisan storage:link
In our team we like to follow a set of rules and have configured our editors to read the phpcs.xml file from the root of our projects.
curl https://git.customd.com/custom-d/linters/-/raw/master/phpcs.xml -o phpcs.xml
Now for some core packages
fin composer require doctrine/dbal laravel/fortify laravel/sanctum
fin artisan vendor:publish --provider="Laravel\Fortify\FortifyServiceProvider"
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
fin composer require --dev imanghafoori/laravel-microscope squizlabs/php_codesniffer beyondcode/laravel-self-diagnosis barryvdh/laravel-ide-helper nunomaduro/phpinsights roave/security-advisories:dev-latest
fin artisan vendor:publish --provider="NunoMaduro\PhpInsights\Application\Adapters\Laravel\InsightsServiceProvider"
fin artisan vendor:publish --provider=BeyondCode\\SelfDiagnosis\\SelfDiagnosisServiceProvider
You may need to edit the config/self-diagnosis file in order to remove the en_us language flag dependant on your setup (also composer 1 / 2 changes waiting on being merged in on the self-diagnosis package)
Add the following to your .gitignore file
.phpstorm.meta.php
_ide_helper.php
cghooks.lock
now you can do your first run:
fin exec vendor/bin/phpcbf --standard=./phpcs.xml -p
fin exec vendor/bin/phpcs --standard=./phpcs.xml -p
fin exec php artisan insights --no-interaction --min-quality=80 --min-complexity=80 --min-architecture=80 --min-style=80
I tend to edit my composer json file and in the scripts add the following to make my life easy (especially for CI Pipelines to run tests etc)
"scripts": {
"post-autoload-dump": [
"Illuminate\\Foundation\\ComposerScripts::postAutoloadDump",
"@php artisan package:discover --ansi",
"@php artisan ide-helper:generate",
"@php artisan ide-helper:meta"
],
"post-root-package-install": [
"@php -r \"file_exists('.env') || copy('.env.example', '.env');\""
],
"post-create-project-cmd": [
"@php artisan key:generate --ansi"
],
"lint:check": "phpcs --standard=./phpcs.xml -p",
"lint:fix": "phpcbf --standard=./phpcs.xml -p",
"test": "@php artisan test --parallel",
"standards": [
"@composer lint:check",
"@php artisan self-diagnosis",
"@php artisan insights --no-interaction --min-quality=80 --min-complexity=80 --min-architecture=80 --min-style=80 --no-interaction",
"@composer tests"
]
}
Links to the documentation on some of these pacakges
We include doctrine/dbal due to being required for migrations which modify columns
for auth we use Fortify and Sanctum but you can also use: Jetstream or Passport